Sentiment Analysis for Stock Trading: A Hands-On Tutorial Using LLMs – Part 1: Predicting Stock Price Movements with FinBert
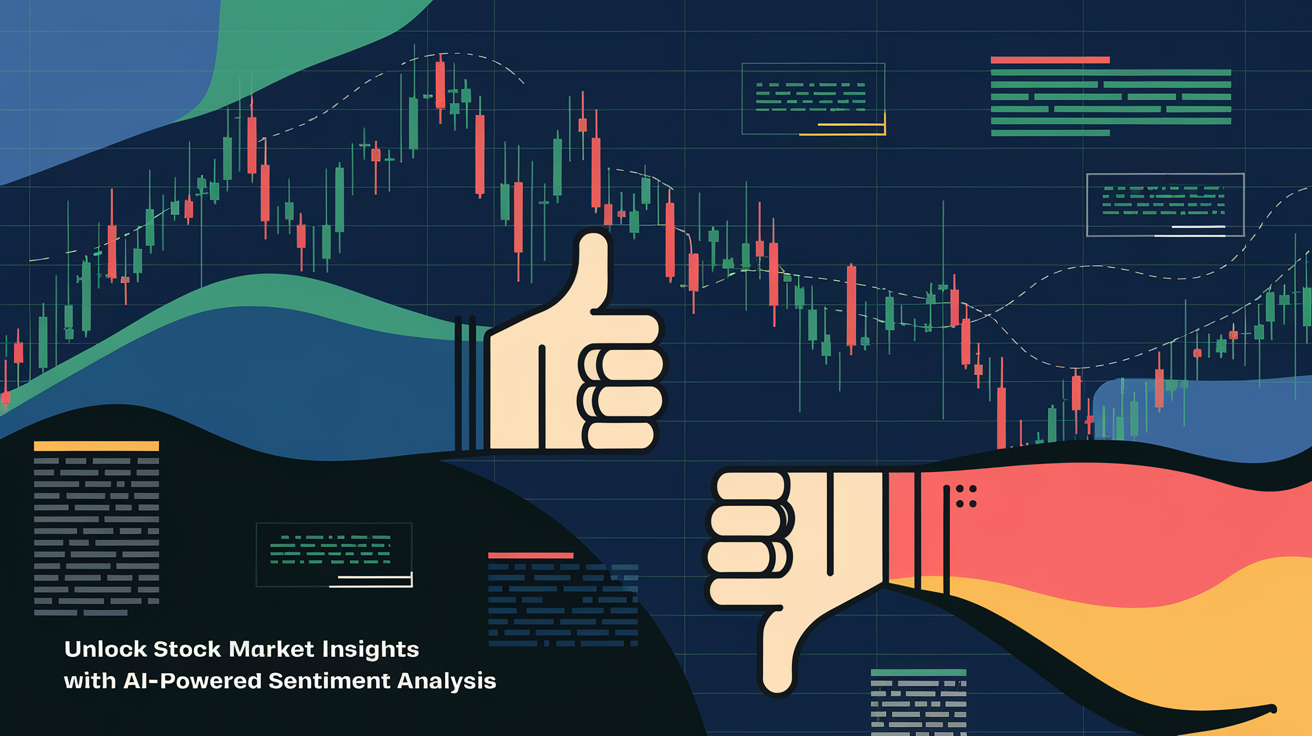
Sentiment Analysis for Stock Trading: A Hands-On Tutorial Using LLMs – Part 1: Predicting Stock Price Movements with FinBert
Welcome to an exciting journey into the world of AI-powered trading!
In this blog, we’ll explore how Large Language Models (LLMs), like FinBert, can read financial news and help predict stock price movements.
In this blog, we’ll explore how Large Language Models (LLMs), like FinBert, can read financial news and help predict stock price movements.
This is Part 1 of a two-part series.
Here, we’ll focus on sentiment analysis
—a way to understand if news is positive, negative, or neutral
—and use it for stock price prediction. In Part 2, we’ll dive into building AI agents for automated trading.
Continue to Part 2: Building AI Agents for Algorithmic Trading.
This tutorial is perfect for traders, developers, and finance enthusiasts who want to use AI in a practical way. We’ll guide you step by step with easy-to-follow Python code and real-world data. By the end, you’ll have a working trading strategy based on sentiment.
Let’s get started!
Why Sentiment Analysis Matters in Trading
Imagine you’re a trader. You read a positive news headline about a company, like Tesla, and its stock price goes up. Or, you see a negative report, and the price drops. This is sentiment at work—how people feel about a stock based on news.
Sentiment analysis helps us understand news and its impact on stock prices. But reading every headline yourself is impossible. That’s where AI comes in. LLMs, like FinBert, can read thousands of news articles quickly and label them as positive, negative, or neutral. This helps traders spot trends early and make better decisions.
In this tutorial, we’ll learn how to:
- Collect financial news data.
- Clean and prepare the text.
- Use FinBert to analyze sentiment.
- Build a simple trading strategy based on sentiment.
- Test your strategy with historical stock data.
Let’s dive in!
Step-by-Step Tutorial
This section is the heart of the blog. We’ll walk you through each step with clear explanations and code.
Step 1: Data Collection
First, we need data. For this tutorial, we’ll use a free dataset from Kaggle called “Daily Financial News for 6000+ Stocks.” It includes news headlines linked to specific stocks.
Since the dataset is large, we’ll focus on one stock: Tesla (TSLA). Tesla’s news is often exciting and changes a lot, making it a fun example. You can choose any stock you like, but Tesla works great for learning.
Here’s how to load and filter the data using Python:
python
import pandas as pd
news_data = pd.read_csv("financial_news.csv")
tesla_news = news_data[news_data["stock"] == "TSLA"]
External Link: Daily Financial News for 6000+ Stocks Kaggle Dataset
Visual Suggestion: Add a screenshot of the dataset’s first few rows. This helps readers see what the data looks like, with columns like “headline,” “stock,” and “date.”
Step 2: Text Preprocessing
News headlines can be messy. They have punctuation, random words, and uppercase letters. To make them readable for our AI model, we need to clean them.
We’ll do three things:
- Tokenize: Break the text into words.
- Remove stop words: Words like “the,” “and,” or “is” that don’t add meaning.
- Lowercase: Make all words lowercase for consistency.
Here’s the code using Python’s NLTK library:
python
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
stop_words = set(stopwords.words("english"))
tesla_news["cleaned_text"] = tesla_news["headline"].apply(
lambda x: " ".join([word.lower() for word in word_tokenize(x) if word.lower() not in stop_words])
)
For example, a headline like “Tesla Announces New Factory!” becomes “tesla announces new factory” after cleaning.
Visual Suggestion: Add a “before and after” table. Show a raw headline (e.g., “Tesla Announces New Factory!”) and its cleaned version (e.g., “tesla announces new factory”). This makes it easy to understand the process.
Step 3: Implementing FinBert for Sentiment Analysis
Now, the fun part! We’ll use FinBert, a special LLM built for financial text. It can read headlines and decide if they’re positive, negative, or neutral.
FinBert is available through Hugging Face’s Transformers library, which makes it easy to use.
Here’s how to load FinBert and analyze the headlines:
python
from transformers import BertTokenizer, BertForSequenceClassification
tokenizer = BertTokenizer.from_pretrained("yiyanghkust/finbert-tone")
model = BertForSequenceClassification.from_pretrained("yiyanghkust/finbert-tone")
def get_sentiment(text):
inputs = tokenizer(text, return_tensors="pt", truncation=True, padding=True, max_length=512)
outputs = model(**inputs)
sentiment = outputs.logits.argmax().item() # 0: negative, 1: neutral, 2: positive
return sentiment - 1 # Scale to -1, 0, 1
tesla_news["sentiment"] = tesla_news["cleaned_text"].apply(get_sentiment)
This code labels each headline with a sentiment score:
- -1: Negative
- 0: Neutral
- 1: Positive
For example, “Tesla reports record profits” might get a +1 (positive), while “Tesla faces lawsuit” might get a -1 (negative).
External Link: FinBert GitHub Repository
Visual Suggestion: Add a bar chart showing the distribution of sentiment scores. For example, it might show 40% positive, 30% neutral, and 30% negative headlines. This helps readers see the results visually.
Step 4: Integrating Sentiment with Trading Strategy
Next, we’ll use the sentiment scores to create a simple trading rule:
- If sentiment is positive (1), buy the stock.
- If sentiment is negative (-1), sell the stock.
- If sentiment is neutral (0), do nothing.
To do this, we need Tesla’s historical stock prices. We’ll use the yfinance library to fetch them.
Here’s the code:
python
import yfinance as yf
tesla_prices = yf.download("TSLA", start="2022-01-01", end="2023-01-01")
merged_data = tesla_news.merge(tesla_prices, left_on="date", right_index=True)
merged_data["signal"] = merged_data["sentiment"].apply(lambda x: 1 if x > 0 else (-1 if x < 0 else 0))
This creates a “signal” column:
- 1: Buy
- -1: Sell
- 0: Hold
For example, if a headline has a +1 sentiment, the signal is 1 (buy). If it has a -1 sentiment, the signal is -1 (sell).
Visual Suggestion: Add a line chart showing Tesla’s stock price over time. Mark buy signals (green dots) and sell signals (red dots) on the chart. This helps readers see how sentiment connects to price changes.
Step 5: Backtesting the Strategy
Now, let’s test our strategy to see if it would have made money. This is called backtesting.
We’ll calculate the returns based on our buy/sell signals and plot the results.
Here’s the code:
python
import matplotlib.pyplot as plt
merged_data["returns"] = merged_data["Close"].pct_change() * merged_data["signal"].shift(1)
merged_data["cumulative_returns"] = (1 + merged_data["returns"]).cumprod()
plt.plot(merged_data.index, merged_data["cumulative_returns"])
plt.title("Cumulative Returns of Sentiment-Based Strategy")
plt.show()
This shows a graph of how your strategy performed over time. If the line goes up, your strategy made money. If it goes down, you might need to tweak your approach.
Visual Suggestion: Add the actual plot generated by the code. Show the cumulative returns over time. This helps readers see if the strategy worked.
Ethical Considerations
AI in trading isn’t perfect. It has risks we need to think about. For example:
- Bias: If the news data is biased, your model might make unfair decisions. For instance, if all news comes from one source, it might not tell the full story.
- Market Impact: If many traders use similar AI strategies, it could cause big price swings in the market.
To be responsible:
- Use diverse data sources to avoid bias.
- Regularly check your model’s outputs to make sure they make sense.
Remember, AI is a tool, not a magic solution. Always trade wisely and don’t rely only on AI.
Conclusion and Teaser for Blog 2
You’ve just built a sentiment-based trading strategy using AI! Here’s what we covered:
- Collecting and cleaning financial news data.
- Using FinBert to analyze sentiment.
- Creating a simple trading rule based on sentiment.
- Testing your strategy with backtesting.
Now, imagine taking this further—automating your trades with AI agents. That’s what we’ll explore in Part 2: Building AI Agents for Algorithmic Trading. Stay tuned!
Continue to Part 2: Building AI Agents for Algorithmic Trading
Try the code yourself and experiment with different stocks.