Mastering Interactive Brokers( IBKR) API Integration with Python: A Developer’s Guide
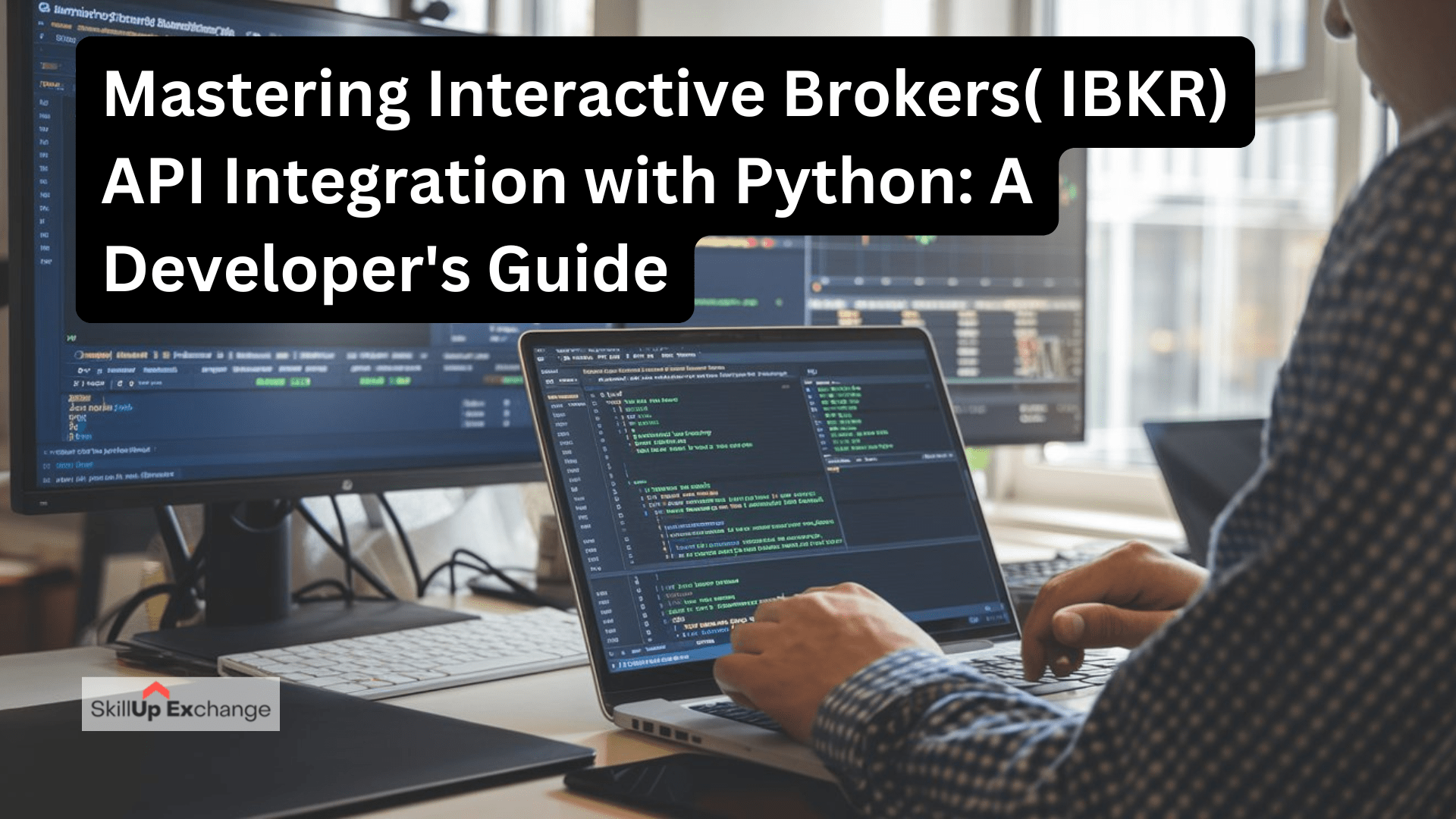
Have you ever wanted to automate your trading strategies or build a custom trading platform? This guide will introduce you to integrating Interactive Brokers (IBKR) with Python, a tool that brings immense potential for algorithmic trading and portfolio management.
Interactive Brokers stands out for its robust API, which empowers developers to build seamless automated trading solutions. From quants to data scientists, this guide simplifies how to connect and use IBKR’s API through Python in a humanized and logical tone, focusing on practical implementation.
Why Choose Interactive Brokers API?
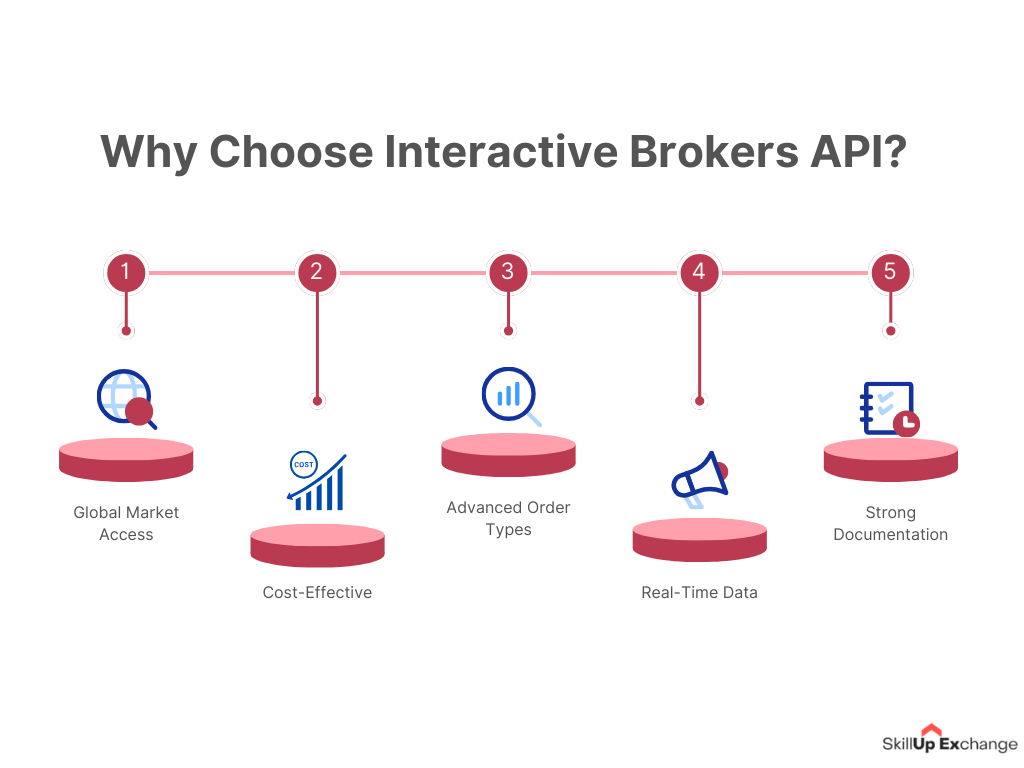
Before diving into integration, consider why IBKR’s API is a standout choice:
- Global Market Access: Trade across 135+ worldwide markets.
- Cost-Effective: Enjoy low commission rates and no API fees.
- Advanced Order Types: Support for over 60 types of orders.
- Real-Time Data: Access accurate and live market data.
- Strong Documentation: Comprehensive API documentation with an active developer community.
By providing these features, Interactive Brokers ensures that you have the right tools to make your trading strategies more effective and streamlined.
Getting Started: Setting Up Your Environment
Prerequisites
To begin, you’ll need the following:
- An Interactive Brokers account (a paper trading account works too).
- Python 3.7 or higher installed on your machine.
- IBKR Trader Workstation (TWS) or IB Gateway.
- Basic Python programming knowledge.
These foundational components ensure a smooth start to using IBKR’s API.
Initial Setup
Begin by installing essential Python packages. Use the following command:
pip install ib_insync pandas numpy
For this guide, we recommend ib_insync
because it offers a user-friendly Python interface and better error handling compared to the official IB API.
Establishing Your First Connection
Step 1: Launch IBKR Trader Workstation
Ensure your Trader Workstation or IB Gateway is running and API access is enabled. To enable API access, follow these steps:
- Open the Trader Workstation.
- Navigate to Edit > Global Configuration.
- Under API > Settings, check the box for “Enable ActiveX and Socket Clients.”
- Set the trusted IP address (leave it as 127.0.0.1 for local use).
Step 2: Writing a Python Script
Now, let’s write a basic Python script to establish the connection:
from ib_insync import *
# Connect to IBKR
ib = IB()
ib.connect('127.0.0.1', 7497, clientId=1)
# Check connection status
if ib.isConnected():
print("Connection Successful!")
else:
print("Connection Failed.")
This script initializes a connection to the Trader Workstation running locally on your computer. The clientId
ensures multiple applications do not conflict.
Step 3: Testing the Connection
Run your script and verify the output. If successful, you should see “Connection Successful!” printed to your console.
Retrieving Market Data
Subscribing to Market Data
To retrieve live market data, you need to subscribe to a ticker. Use the following script:
# Subscribe to market data
tsla_contract = Stock('TSLA', 'SMART', 'USD')
tsla_data = ib.reqMktData(tsla_contract)
print(f"TSLA Price: {tsla_data.last}")
This script fetches the real-time price of Tesla’s stock. Ensure you have the necessary market data subscriptions enabled on your IBKR account.
Placing Your First Trade
Creating an Order
Placing an order involves defining a contract and the order details. Here’s a simple example:
# Define the contract and order
contract = Stock('AAPL', 'SMART', 'USD')
order = MarketOrder('BUY', 10)
# Place the order
trade = ib.placeOrder(contract, order)
print("Order placed:", trade)
This script places a market order to buy 10 shares of Apple stock. Replace “BUY” with “SELL” to place a sell order.
Error Handling and Debugging
Common Issues
- Connection Errors: Ensure your Trader Workstation or IB Gateway is running and API access is enabled.
- Invalid Tickers: Double-check the ticker symbol and exchange.
- Market Data Subscription: Confirm you have the appropriate market data subscriptions.
Logging
Enable logging in your script to track issues:
import logging
logging.basicConfig(level=logging.DEBUG)
This will display detailed logs for debugging.
Advanced Features of IBKR API
Algorithmic Trading
With IBKR’s API, you can implement sophisticated trading algorithms. Leverage libraries like numpy
and pandas
to analyze market trends and make data-driven decisions.
Portfolio Management
Retrieve and analyze your portfolio using:
portfolio = ib.positions()
for pos in portfolio:
print(pos)
This script lists all open positions in your portfolio.
Conclusion
Integrating Interactive Brokers API with Python unlocks a world of possibilities for traders and developers. From automating trades to building custom analytics tools, the IBKR API is a powerful asset. To further elevate your algorithmic trading skills, be sure to check out our previous blog, “Why FinGPT is Redefining Python Algo Trading for Professionals.” By understanding how FinGPT is transforming the landscape, you’ll be better equipped to explore advanced capabilities of the IBKR API. Following this guide is just the beginning of your journey toward mastering automated trading with Python.