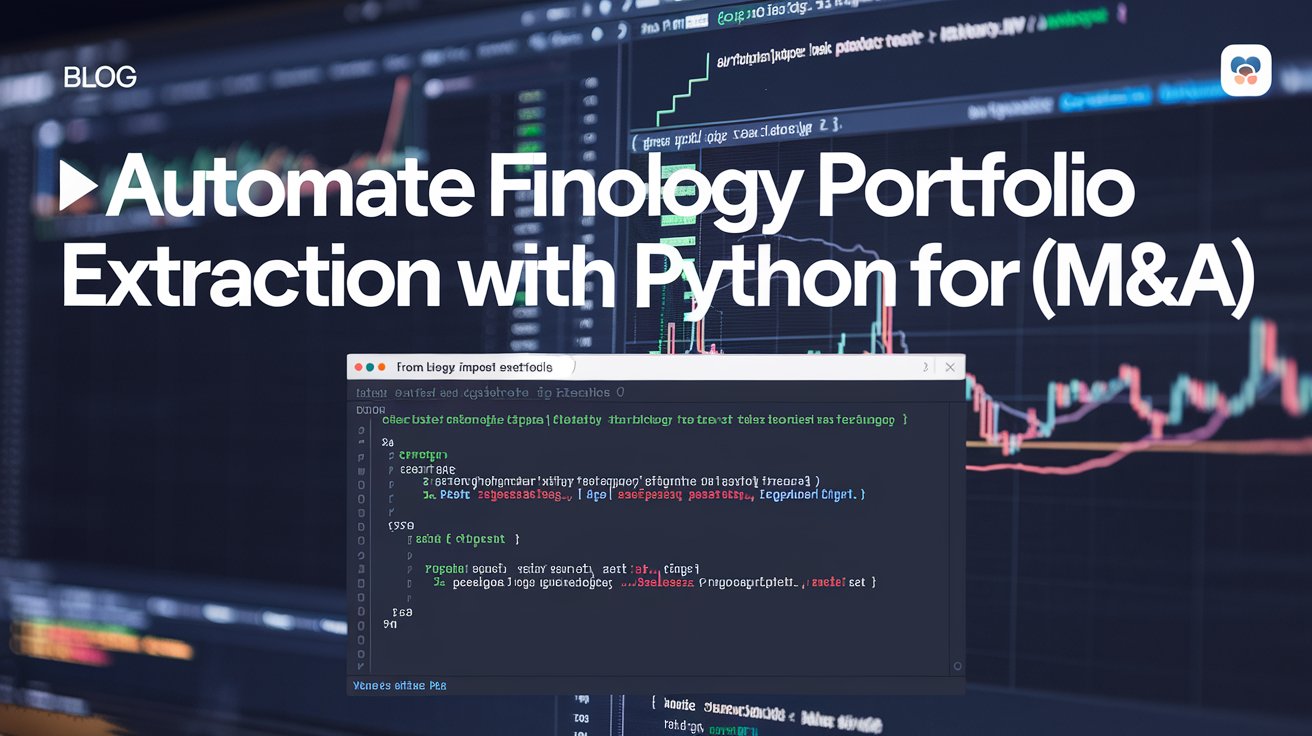
Picture this scenario: You’re an M&A professional racing against the clock to close a high-value acquisition. Time is short, competition is fierce, and your team can’t afford costly mistakes. Yet you find yourself bogged down by repetitive, manual tasks—like scraping portfolio data from Finology—that eat into your valuable time. In a process where every minute counts, even the smallest inefficiencies can mean missed opportunities or miscalculated risks.
Mergers and Acquisitions (M&A) are high-stakes endeavors critical for business growth, market expansion, and strategic advantage. However, the complexity of M&A—encompassing due diligence, negotiations, and post-merger integration—can strain resources and create opportunities for errors. From analyzing target companies’ financial portfolios to integrating massive amounts of data, M&A professionals require robust, efficient tools that help them move swiftly and decisively.
This blog post explores how you can automate portfolio data extraction from Finology using Python, empowering M&A teams to reduce manual errors, stay on top of real-time data, and ultimately make better, faster decisions. By the end of this guide, you’ll understand the key benefits of automation, practical steps to implement it, and best practices to ensure data integrity.
Why M&A Professionals Need Automation
M&A deals hinge on a few core imperatives: speed, accuracy, and foresight. Let’s dive into the main pain points that make automation a game-changer in this space.
- Time Constraints
- Traditional M&A workflows can be slow and labor-intensive, especially when manually collecting and consolidating data from multiple sources.
- In a competitive market, time is critical: taking too long to finalize a deal can result in lost opportunities or a competitive disadvantage.
- Risk of Manual Errors
- Human error is almost inevitable when transcribing data or performing repetitive tasks under pressure.
- Even small inaccuracies in financial calculations can jeopardize compliance or lead to poor strategic decisions.
Automation tackles these challenges by removing the tedium of manual data collection and minimizing human error. Next, we’ll look at how technologies like AI and Robotic Process Automation (RPA) are reshaping the M&A landscape.
Benefits of Automating Portfolio Data Extraction
Automation addresses these challenges head-on by introducing:
- Speed
- Automated scripts rapidly gather data from multiple sources, freeing up your team to interpret and act on that data rather than hunt for it.
- When deals need to move fast, this speed can be the difference between winning and losing a valuable opportunity.
- Reliability
- Standardized, automated workflows reduce variability. The same process runs every time, ensuring data is consistently captured in the correct format.
- This predictability helps build trust in your data, which is essential for making sound M&A decisions.
- Real-Time Updates
- Automated systems can pull data at scheduled intervals (daily, hourly, or even more frequently), providing continuous access to the latest figures.
- In rapidly fluctuating markets, real-time data can be a game-changer for deal negotiations and valuations.
Prerequisites & Environment Setup
Before diving in, ensure you have the following:
- Python 3.8 or Higher
- Libraries:
requests
,beautifulsoup4
,selenium
, and (optionally)investpy
if you want to pull data from Investing.com.
Open your terminal and run:
pip install requests beautifulsoup4 selenium investpy
Virtual Environment (Recommended)
It’s best practice to use a virtual environment to isolate dependencies:
# Create a directory for your project
mkdir portfolio_data_extraction && cd portfolio_data_extraction
# Create and activate a virtual environment
python3 -m venv env
source env/bin/activate # For Mac/Linux
.\env\Scripts\activate # For Windows
# Install the libraries
pip install requests beautifulsoup4 selenium investpy
Step-by-Step Tutorial: Automating Data Extraction from Finology
Below is a concise tutorial to help you quickly implement an automated data extraction pipeline.
Step 1: Environment Setup
- Project Directory: Make a dedicated folder for your project.
- Virtual Environment: Use Python’s
venv
to keep your dependencies organized. - Library Installation: As described above, install all necessary libraries.
Step 2: Accessing Finology Data
Depending on Finology’s offering, you may have:
- API Access: The most direct and reliable method if Finology provides it.
- Web Scraping: If no official API is available, you’ll need to scrape directly from their website.
For the sake of illustration, let’s assume we must log into Finology and extract data. This is where Selenium shines.
Step 3: Extracting Portfolio Information
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
# Set up the WebDriver
driver = webdriver.Chrome() # Make sure you have ChromeDriver installed
# Navigate to Finology login
driver.get("https://finology.com/login")
# Input credentials
username = driver.find_element(By.NAME, "username")
password = driver.find_element(By.NAME, "password")
username.send_keys("your_username")
password.send_keys("your_password")
password.send_keys(Keys.RETURN)
# Wait for login
time.sleep(5) # Adjust based on your connection
# Go to portfolio page
driver.get("https://finology.com/portfolio")
# Extract portfolio data
portfolio_data = driver.find_elements(By.CLASS_NAME, "portfolio-item")
for item in portfolio_data:
print(item.text)
driver.quit()
This sample script demonstrates how to navigate to Finology, log in, and scrape data. Adjust CSS selectors (CLASS_NAME
, NAME
, etc.) according to the actual HTML structure of the website.
Step 4: Data Transformation & Storage
After extracting raw data, you can parse and structure it using pandas:
import pandas as pd
# Example data
data = {
"Stock": ["AAPL", "GOOGL", "AMZN"],
"Shares": [10, 5, 2],
"Price": [150.00, 2800.00, 3400.00]
}
df = pd.DataFrame(data)
# Save as CSV or Excel
df.to_csv("portfolio_data.csv", index=False)
df.to_excel("portfolio_data.xlsx", index=False)
This final step helps you store data in a format that’s easy to integrate into financial models or other analytical tools.
Putting Your Data to Work in M&A
Feeding Extracted Data into Financial Models
- M&A Financial Modeling
- Pro Forma Financials: Combine acquiring and target company financials to forecast combined performance.
- Synergy Estimates: Quantify cost savings and revenue enhancements due to the merger.
- Accretion/Dilution Analysis: Assess whether the deal will increase or decrease the EPS of the acquirer.
- Dashboards for Real-Time Evaluation
- Use tools like Tableau or Power BI to visualize revenue growth, cost synergies, and market performance indicators in real time.
- Set up live connections to your extracted data so that dashboards automatically update as new information becomes available.
Keeping Data Fresh Automatically
- Cron Jobs (Linux/Mac)
Schedule daily updates by adding a line like this to your crontab0 8 * * * /usr/bin/python /path/to/your_script.py
- Task Scheduler (Windows)
Set up a basic task to run your script every morning at 8 AM, ensuring your data is always up-to-date when you start your day. - Cloud Solutions
Deploy your scripts to AWS Lambda or Google Cloud Functions to offload the infrastructure overhead. This can be especially helpful for larger operations or for teams without dedicated on-prem servers.
Troubleshooting & Best Practices
Common Pitfalls
- Authentication Hurdles: Complex login pages require robust scripting. Consider using Selenium for multi-step login forms.
- Site Structure Changes: Websites update often. Regularly test your scraping scripts to catch breaks early.
- Rate Limits: Avoid spamming the server. Implement polite scraping by adding delays (
time.sleep()
) or random intervals.
Handling Large Datasets
- Batch Processing: If the dataset is huge, break it into smaller chunks to prevent memory overload.
- Caching: Cache HTML pages locally to minimize redundant requests, improving efficiency.
- Proxies: Consider rotating IP addresses if you’re making numerous requests to avoid potential IP blocking.
Data Integrity
- Validation: Compare scraped data against known benchmarks or smaller manual samples to confirm accuracy.
- Monitoring: Use logging and alerts to track your scraper’s performance.
- Error Handling: Implement try-except blocks to gracefully manage unresponsive pages or missing elements.
Conclusion
Automating portfolio data extraction can drastically improve how M&A professionals manage and analyze financial information—freeing you to focus on strategy, mitigating the risk of errors, and swiftly responding to market dynamics. By harnessing Python’s versatile ecosystem, you’ll:
- Boost Efficiency: Eliminate the tedium of manual data scraping.
- Enhance Accuracy: Standardize and validate data, an essential factor in high-stakes deals.
- Stay Agile: Maintain real-time visibility into your portfolios, vital when time is of the essence.
- Scale Seamlessly: Handle growing deal volumes without linear increases in manual workload.
Take Your M&A Skillset Even Further
To continue accelerating your success in the dynamic M&A landscape, consider exploring these additional resources and opportunities:
- Further Reading – 7 Strategies for Improving Generative AI Accuracy in M&A
Delve into the cutting edge of AI-driven dealmaking. Discover how generative AI can refine your M&A analysis by improving precision in everything from valuation models to risk assessments. - Cohort-Based Course – Generative AI-Driven Insights for M&A, Venture Capital, and Private Equity Professionals
Elevate your dealmaking expertise by enrolling in this practical, cohort-based course. You’ll learn how to leverage generative AI for deeper insights, faster due diligence, and more confident decision-making in M&A, venture capital, and private equity contexts.
By combining automated portfolio data extraction with advanced AI strategies, you’ll forge a powerful toolkit that keeps you competitive in an ever-evolving market. Start implementing these approaches today, and watch as your deals become faster, more accurate, and ultimately more successful.
Is web scraping Finology data legal?
Scraping Finology data is typically permissible if you only access public data, comply with Finology’s Terms of Service, avoid restricted or personal information, and adhere to all applicable privacy laws
Do I need advanced coding skills to automate portfolio data extraction?
Basic Python knowledge is usually enough. Many libraries like Selenium and Beautiful Soup are beginner-friendly, and numerous online tutorials can guide you step by step.
What if Finology changes its website layout?
Regularly monitor your script’s performance and implement error handling. Minor HTML changes can break your scraper, so be prepared to update the affected selectors or methods.