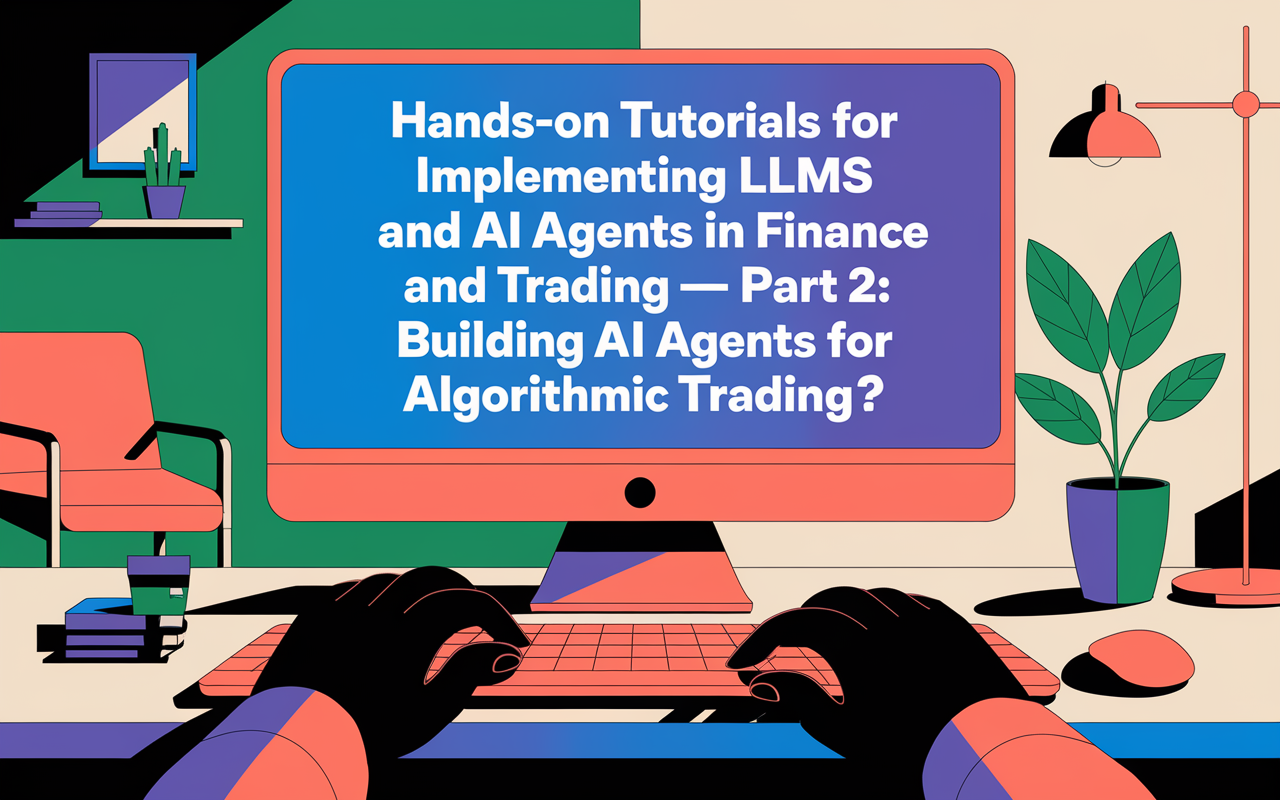
Hands-On Tutorials for Implementing LLMs and AI Agents in Finance and Trading – Part 2: Building AI Agents for Algorithmic Trading
Hands-On Tutorials for Implementing LLMs and AI Agents in Finance and Trading – Part 2: Building AI Agents for Algorithmic Trading
Welcome back to our blog series on using AI in finance and trading! In Part 1, we explored how to use Large Language Models (LLMs) to analyze news headlines and gauge market sentiment.
Now, in Part 2, we’re stepping things up. We’ll build an AI agent that uses machine learning to make trading decisions, combining sentiment analysis from Part 1 with technical indicators to predict stock price movements. Plus, we’ll test our strategy using Backtrader, a powerful Python library for backtesting trading ideas.
By the end of this guide, you’ll have your own AI-powered trading bot. Whether you’re a trader, a coder, or just curious about AI, this tutorial is designed to be easy to follow—no prior experience required. Let’s dive in!
Why AI Agents for Trading?
Imagine having a tireless assistant who analyzes mountains of data—stock prices, news, trends—and spots opportunities you might miss. That’s what an AI agent does in algorithmic trading. These agents use machine learning to learn from past data, make predictions, and act fast, all without the emotional baggage humans sometimes bring to trading.
In this post, we’ll create an AI agent using a random forest classifier, a simple yet effective machine learning model. It’ll take inputs like stock prices, technical indicators, and sentiment scores to decide whether to buy or sell. Then, we’ll use Backtrader to see how it performs with historical data.
What You’ll Learn
Here’s the roadmap for this ~2000-word adventure:
- Collecting and Preparing Stock Data: We’ll grab historical stock prices and add technical indicators.
- Training a Machine Learning Model: We’ll build a random forest to predict buy or sell signals.
- Backtesting with Backtrader: We’ll run a simulation to see how our strategy holds up.
Don’t worry if this sounds intimidating—we’ll break it down into bite-sized steps!
Prerequisites
Before we begin, you’ll need a few tools. Don’t panic—they’re all free and easy to set up:
- Python: The programming language we’ll use (version 3.8+ recommended).
- Libraries: Install these with pip:
- yfinance (for stock data)
- ta (for technical indicators)
- scikit-learn (for machine learning)
- backtrader (for backtesting)
- matplotlib (for optional charts)
- Setup Tip: Use a virtual environment to keep things tidy:bash
python -m venv trading_env source trading_env/bin/activate # On Windows: trading_env\Scripts\activate pip install yfinance ta scikit-learn backtrader matplotlib
Got everything? Great—let’s move on!
Step 1: Collecting and Preparing Stock Data
Our AI agent needs data to learn from. We’ll use Tesla (TSLA) stock data from 2020 to 2023 as our example. We’ll also calculate some technical indicators—tools traders love for spotting trends—and add sentiment scores from Part 1.
What Are Technical Indicators?
Think of technical indicators as cheat codes for understanding stock charts:
- Simple Moving Average (SMA): The average price over a set time (e.g., 20 days) to smooth out noise.
- Relative Strength Index (RSI): Measures if a stock is overbought (above 70) or oversold (below 30).
- MACD: Shows momentum by comparing two moving averages.
These, plus sentiment, will give our agent a full picture of what’s happening.
Fetching the Data
We’ll use yfinance to grab Tesla’s data and ta to compute indicators. Here’s the code:
python
import yfinance as yf
import pandas as pd
from ta.trend import SMAIndicator, MACD
from ta.momentum import RSIIndicator
# Fetch Tesla stock data
stock = yf.download('TSLA', start='2020-01-01', end='2023-12-31')
data = stock[['Close', 'Volume']].copy()
# Add technical indicators
data['SMA_20'] = SMAIndicator(data['Close'], window=20).sma_indicator() # 20-day SMA
data['RSI'] = RSIIndicator(data['Close'], window=14).rsi() # 14-day RSI
macd = MACD(data['Close'])
data['MACD'] = macd.macd() # MACD line
# Add sentiment scores (placeholder—replace with Part 1 data)
data['Sentiment'] = 0
# Remove rows with missing data
data = data.dropna()
print(data.head())
What’s Going On?
- We grab Tesla’s closing prices and volume from 2020 to 2023.
- We calculate a 20-day SMA, 14-day RSI, and MACD.
- We add a dummy column for sentiment (set to 0). If you have real sentiment scores from Part 1, plug them in here!
- We drop rows with missing values to keep our data clean.
This prepped data is the foundation of our AI agent.
Step 2: Building the Machine Learning Model
Time to test our AI agent! We’ll use Backtrader to simulate trades and see how much money we’d make (or lose).
What’s Backtesting?
Backtesting lets you “play” your trading strategy on past data. It’s like a rehearsal—see what works before risking real cash.
Setting Up Backtrader
First, we prepare our data for Backtrader:
python
import backtrader as bt
# Stock data feed for 2023
data_feed = bt.feeds.PandasData(dataname=stock['2023-01-01':'2023-12-31'])
# Predictions feed
pred_feed = bt.feeds.PandasData(dataname=predictions, datetime=None, open=-1, high=-1, low=-1, close='Signal')
What’s Going On?
- We create two feeds: one for Tesla’s 2023 prices, one for our model’s signals.
Creating the Strategy
Our rules are simple:
- Signal = 1? Buy 100 shares.
- Signal = 0 and we own shares? Sell them all.
Here’s the code:
python
class MLStrategy(bt.Strategy):
def __init__(self):
self.predictions = self.datas[1].close # Predictions feed
self.order = None
def next(self):
if self.order:
return
signal = self.predictions[0] if len(self.predictions) > 0 else 0
if not self.position: # Not in market
if signal == 1:
self.order = self.buy(size=100) # Buy 100 shares
else: # In market
if signal == 0:
self.order = self.sell(size=self.position.size) # Sell all
def notify_order(self, order):
if order.status in [order.Completed]:
self.order = None
What’s Happening?
- Each day, we check the signal.
- If it’s 1 and we’re not in the market, we buy.
- If it’s 0 and we’re holding, we sell.
Running the Backtest
Let’s simulate with $100,000 starting cash:
python
# Set up Backtrader
cerebro = bt.Cerebro()
cerebro.addstrategy(MLStrategy)
cerebro.adddata(data_feed) # Stock data
cerebro.adddata(pred_feed) # Predictions
cerebro.broker.setcash(100000.0)
cerebro.addanalyzer(bt.analyzers.DrawDown, _name='drawdown')
cerebro.addanalyzer(bt.analyzers.TradeAnalyzer, _name='trades')
# Run it
print('Starting Portfolio Value: %.2f' % cerebro.broker.getvalue())
results = cerebro.run()
print('Final Portfolio Value: %.2f' % cerebro.broker.getvalue())
# Get metrics
drawdown = results[0].analyzers.drawdown.get_analysis()
trades = results[0].analyzers.trades.get_analysis()
print(f'Max Drawdown: {drawdown.max.drawdown:.2f}%')
print(f'Total Trades: {trades.total.total}')
print(f'Winning Trades: {trades.won.total}')
What’s Going On?
- We start with $100,000 and run the strategy on 2023 data.
- We track performance: final value, biggest loss (drawdown), and trade stats.
Seeing the Results (Optional)
Want a visual? Add this:
python
cerebro.plot()
This shows your portfolio’s growth over time—pretty cool, right?
Step 3: Implementing and Backtesting the Strategy with Backtrader
Next, we’ll train a random forest classifier to predict whether Tesla’s stock price will rise (buy) or not (hold/sell).
What’s a Random Forest?
Picture a random forest as a group of friends voting on a decision. Each “tree” looks at the data—prices, indicators, sentiment—and makes a guess. The group then picks the most popular answer. It’s a straightforward way to handle lots of inputs and make solid predictions.
Defining the Goal
We want our model to predict if tomorrow’s price will be higher than today’s. If yes, it’s a “buy” signal (1); if no, it’s a “no buy” (0). Here’s how we set that up:
python
# Create target: 1 if next day’s close is higher, else 0
data['Target'] = (data['Close'].shift(-1) > data['Close']).astype(int)
data = data[:-1] # Drop last row (no next day)
# Features (inputs) and target (output)
features = ['Close', 'Volume', 'SMA_20', 'RSI', 'MACD', 'Sentiment']
X = data[features]
y = data['Target']
What’s Happening?
- We make a “Target” column: 1 if the next day’s price is higher, 0 if not.
- We trim the last row since it has no “next day” to predict.
- Our inputs (features) are the price, volume, indicators, and sentiment.
Training the Model
We’ll train on 2020-2022 data and test on 2023 to see how it handles new info:
python
from sklearn.ensemble import RandomForestClassifier
# Split data: train on 2020-2022, test on 2023
train_data = data['2020-01-01':'2022-12-31']
test_data = data['2023-01-01':'2023-12-31']
X_train = train_data[features]
y_train = train_data['Target']
X_test = test_data[features]
y_test = test_data['Target']
# Train the random forest
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Check accuracy (optional)
accuracy = model.score(X_test, y_test)
print(f'Model Accuracy: {accuracy:.2f}')
What’s Going On?
- We split the data by time: 2020-2022 for training, 2023 for testing.
- We train a random forest with 100 trees (a good default).
- We measure accuracy on the test set, though in trading, profit matters more than precision.
Making Predictions
Now, let’s predict signals for 2023:
python
# Predict signals for 2023
test_data['Signal'] = model.predict(X_test)
predictions = test_data[['Signal']].copy()
print(predictions.head())
What’s Happening?
- The model gives us a 1 (buy) or 0 (no buy) for each day in 2023.
- We save these predictions for our trading strategy.
Results and Insights
Your results will depend on Tesla’s 2023 performance and your sentiment data. Here’s what to check:
- Final Portfolio Value: Did you beat $100,000?
- Max Drawdown: How risky was it?
- Total Trades: Was it too active or just right?
- Winning Trades: Did more trades win than lose?
One big takeaway: Combining machine learning with Backtrader is simpler than it looks. By precomputing predictions, we keep things beginner-friendly. Try tweaking the features or stock—experimenting is half the fun!
Visual Idea: A chart of your equity curve (portfolio value over time) and drawdown would make these results pop.
Conclusion
You did it! You’ve built an AI agent for algorithmic trading. To recap:
- We gathered stock data and added technical indicators.
- We trained a random forest to predict price movements.
- We backtested it with Backtrader to see the results.
This is just the start. Play with different stocks, add more features, or tweak the model. Next time, we’ll tackle real-time trading or dive into advanced AI techniques like reinforcement learning.
Want to take your trading to the next level? Check out our blog AI Agents for Stock Trading: The Future of Investing.
Learn how AI can transform your strategy and keep you ahead in the market!